Python Slots Conflicts With Class Variable
Next Chapter: Classes and Class Creation
In this particular example, the slot class is about 35% faster. Conclusion & Further Reading. Data classes are one of the new features of Python 3.7. With data classes, you do not have to write boilerplate code to get proper initialization, representation, and comparisons for your objects. You have seen how to define your own data classes, as.
Slots
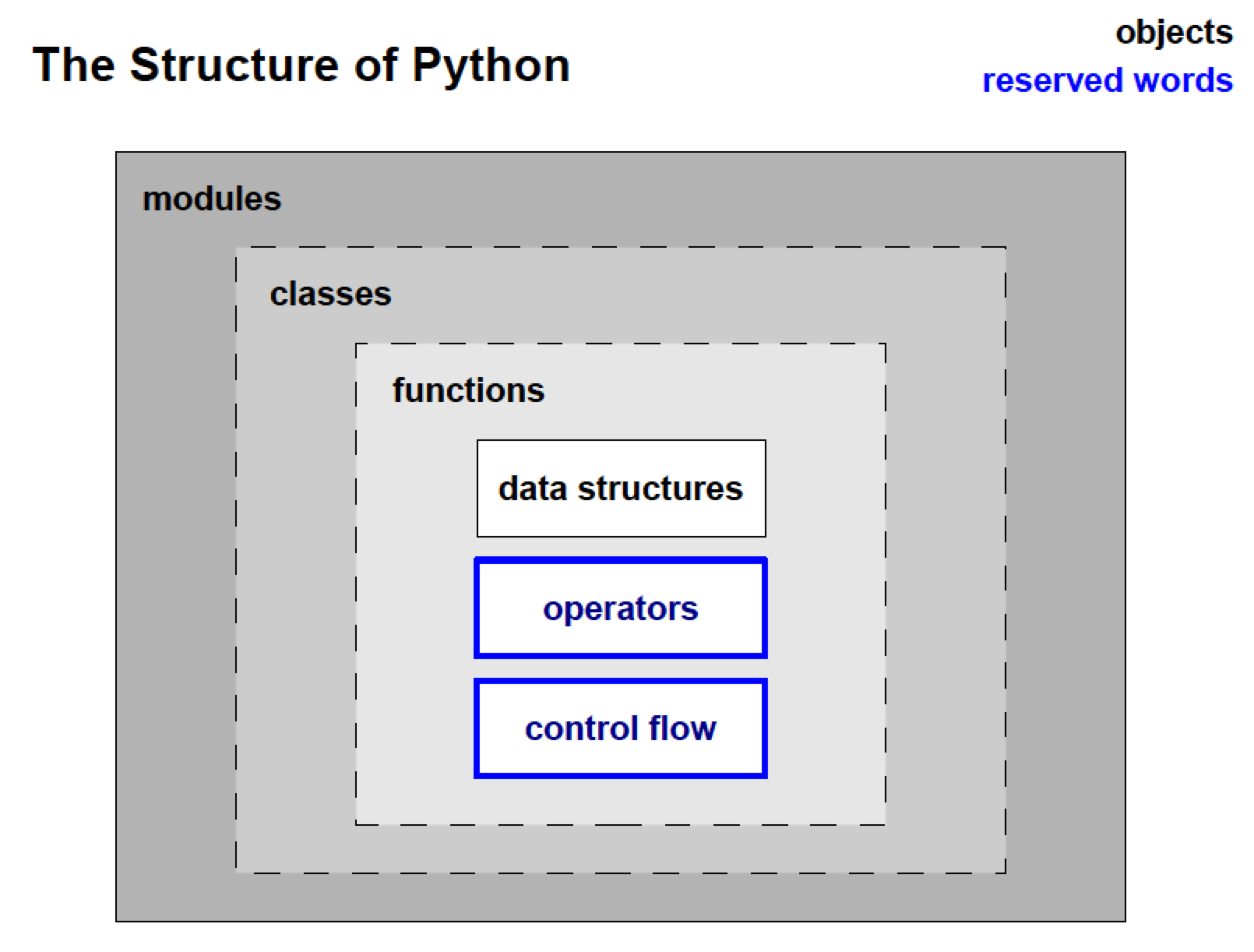
Instead of changing a class variable Python creates a new instance variable with the same name. Hence, the instance variables have precedence over class variables when searching for an attribute value. Mutable class variables. You need to be very careful when working with mutable class variables (e.g., list, set, dictionary). I'm afraid that's not (easily) possible. Using slots implicitly gives classes attributes, so what you are seeing is the effect of toying with that. It's not really possible to pretend there are different attributes on the class than the descriptors. We can: - Silently override slot descrs with attributes (as currently done). In Python 3, this was triggering this error: builtins.ValueError: 'offset' in slots conflicts with class variable In Python 2, if you defined slots and after that defined a property with the same name the slots descriptor was replaced silently. In Python 3 this isn't allowed anymore. I've got conflicts between Python's typing system and slots. Here is a small reproducible example. From typing import TypeVar, Generic, Sequence T = TypeVar('T') class TestGeneric(Sequence.
Avoiding Dynamically Created Attributes
The attributes of objects are stored in a dictionary __dict__
. Like any other dictionary, a dictionary used for attribute storage doesn't have a fixed number of elements. In other words, you can add elements to dictionaries after they are defined, as we have seen in our chapter on dictionaries. This is the reason, why you can dynamically add attributes to objects of classes that we have created so far:

The dictionary containing the attributes of 'a' can be accessed like this:
You might have wondered that you can dynamically add attributes to the classes, we have defined so far, but that you can't do this with built-in classes like 'int', or 'list':
Using a dictionary for attribute storage is very convenient, but it can mean a waste of space for objects, which have only a small amount of instance variables. The space consumption can become critical when creating large numbers of instances. Slots are a nice way to work around this space consumption problem. Instead of having a dynamic dict that allows adding attributes to objects dynamically, slots provide a static structure which prohibits additions after the creation of an instance.
When we design a class, we can use slots to prevent the dynamic creation of attributes. To define slots, you have to define a list with the name __slots__
. The list has to contain all the attributes, you want to use. We demonstrate this in the following class, in which the slots list contains only the name for an attribute 'val'.
If we start this program, we can see, that it is not possible to create dynamically a new attribute. We fail to create an attribute 'new'.

We mentioned in the beginning that slots are preventing a waste of space with objects. Since Python 3.3 this advantage is not as impressive any more. With Python 3.3 Key-Sharing Dictionaries are used for the storage of objects. The attributes of the instances are capable of sharing part of their internal storage between each other, i.e. the part which stores the keys and their corresponding hashes. This helps reducing the memory consumption of programs, which create many instances of non-builtin types.
Next Chapter: Classes and Class Creation
An inner class or nested class is a defined entirely within the body of another class. If an object is created using a class, the object inside the root class can be used. A class can have more than one inner classes, but in general inner classes are avoided.
Related Course:
Python Programming Bootcamp: Go from zero to hero
Inner class example
We create a class (Human) with one inner class (Head).
An instance is created that calls a method in the inner class:
Output:
In the program above we have the inner class Head() which has its own method. An inner class can have both methods and variables. In this example the constructor of the class Human (init) creates a new head object.
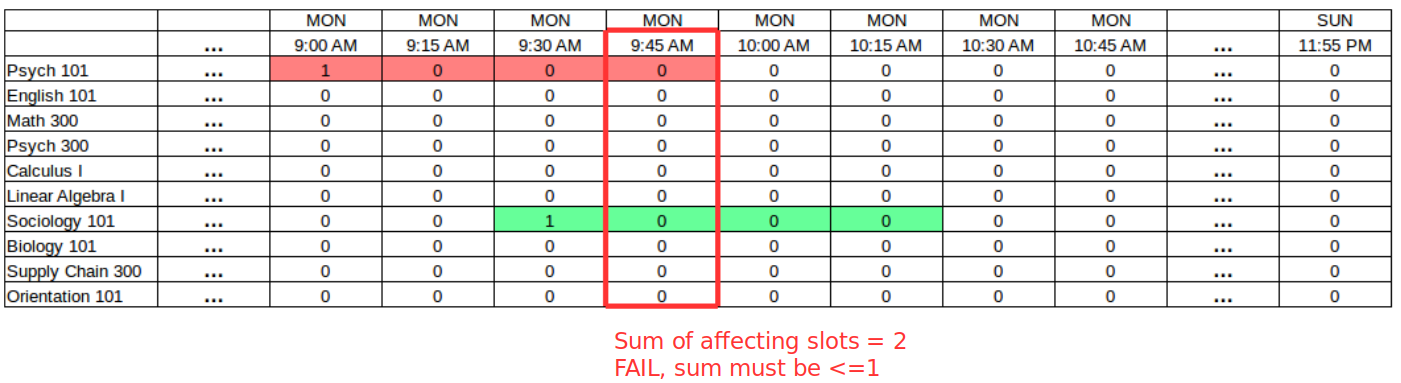
Multiple inner classes
You are by no means limited to the number of inner classes, for example this code will work too:

Python Slots Conflicts With Class Variables
By using inner classes you can make your code even more object orientated. A single object can hold several sub objects. We can use them to add more structure to our programs.
Python Class Member Variable
If you are new to Python programming, I highly recommend this book.